mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-11-11 08:46:16 +02:00
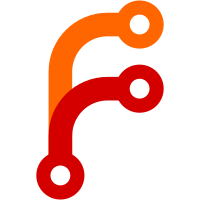
Move clearValue inside Value mkInt instead of setInt mkBool instead of setBool mkString instead of setString mkPath instead of setPath mkNull instead of setNull mkAttrs instead of setAttrs mkList instead of setList* mkThunk instead of setThunk mkApp instead of setApp mkLambda instead of setLambda mkBlackhole instead of setBlackhole mkPrimOp instead of setPrimOp mkPrimOpApp instead of setPrimOpApp mkExternal instead of setExternal mkFloat instead of setFloat Add note that the static mk* function should be removed eventually
56 lines
1.3 KiB
C++
56 lines
1.3 KiB
C++
#include "attr-set.hh"
|
|
#include "eval-inline.hh"
|
|
|
|
#include <algorithm>
|
|
|
|
|
|
namespace nix {
|
|
|
|
|
|
/* Allocate a new array of attributes for an attribute set with a specific
|
|
capacity. The space is implicitly reserved after the Bindings
|
|
structure. */
|
|
Bindings * EvalState::allocBindings(size_t capacity)
|
|
{
|
|
if (capacity > std::numeric_limits<Bindings::size_t>::max())
|
|
throw Error("attribute set of size %d is too big", capacity);
|
|
return new (allocBytes(sizeof(Bindings) + sizeof(Attr) * capacity)) Bindings((Bindings::size_t) capacity);
|
|
}
|
|
|
|
|
|
void EvalState::mkAttrs(Value & v, size_t capacity)
|
|
{
|
|
if (capacity == 0) {
|
|
v = vEmptySet;
|
|
return;
|
|
}
|
|
v.mkAttrs(allocBindings(capacity));
|
|
nrAttrsets++;
|
|
nrAttrsInAttrsets += capacity;
|
|
}
|
|
|
|
|
|
/* Create a new attribute named 'name' on an existing attribute set stored
|
|
in 'vAttrs' and return the newly allocated Value which is associated with
|
|
this attribute. */
|
|
Value * EvalState::allocAttr(Value & vAttrs, const Symbol & name)
|
|
{
|
|
Value * v = allocValue();
|
|
vAttrs.attrs->push_back(Attr(name, v));
|
|
return v;
|
|
}
|
|
|
|
|
|
Value * EvalState::allocAttr(Value & vAttrs, const std::string & name)
|
|
{
|
|
return allocAttr(vAttrs, symbols.create(name));
|
|
}
|
|
|
|
|
|
void Bindings::sort()
|
|
{
|
|
std::sort(begin(), end());
|
|
}
|
|
|
|
|
|
}
|