mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-09-21 08:58:04 +03:00
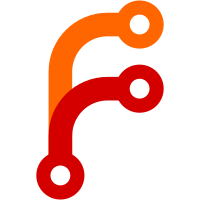
When calling `builtins.readFile` on a store path, the references of that path are currently added to the resulting string's context. This change makes those references the *possible* context of the string, but filters them to keep only the references whose hash actually appears in the string, similarly to what is done for determining the runtime references of a path.
71 lines
1.4 KiB
C++
71 lines
1.4 KiB
C++
#pragma once
|
|
|
|
#include "hash.hh"
|
|
#include "path.hh"
|
|
|
|
namespace nix {
|
|
|
|
std::pair<StorePathSet, HashResult> scanForReferences(const Path & path, const StorePathSet & refs);
|
|
|
|
StorePathSet scanForReferences(Sink & toTee, const Path & path, const StorePathSet & refs);
|
|
|
|
class RefScanSink : public Sink
|
|
{
|
|
StringSet hashes;
|
|
StringSet seen;
|
|
|
|
std::string tail;
|
|
|
|
public:
|
|
|
|
RefScanSink(StringSet && hashes) : hashes(hashes)
|
|
{ }
|
|
|
|
StringSet & getResult()
|
|
{ return seen; }
|
|
|
|
void operator () (std::string_view data) override;
|
|
};
|
|
|
|
class PathRefScanSink : public RefScanSink
|
|
{
|
|
std::map<std::string, StorePath> backMap;
|
|
|
|
PathRefScanSink(StringSet && hashes, std::map<std::string, StorePath> && backMap);
|
|
|
|
public:
|
|
|
|
static PathRefScanSink fromPaths(const StorePathSet & refs);
|
|
|
|
StorePathSet getResultPaths();
|
|
};
|
|
|
|
struct RewritingSink : Sink
|
|
{
|
|
std::string from, to, prev;
|
|
Sink & nextSink;
|
|
uint64_t pos = 0;
|
|
|
|
std::vector<uint64_t> matches;
|
|
|
|
RewritingSink(const std::string & from, const std::string & to, Sink & nextSink);
|
|
|
|
void operator () (std::string_view data) override;
|
|
|
|
void flush();
|
|
};
|
|
|
|
struct HashModuloSink : AbstractHashSink
|
|
{
|
|
HashSink hashSink;
|
|
RewritingSink rewritingSink;
|
|
|
|
HashModuloSink(HashType ht, const std::string & modulus);
|
|
|
|
void operator () (std::string_view data) override;
|
|
|
|
HashResult finish() override;
|
|
};
|
|
|
|
}
|