mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2025-02-14 14:17:18 +02:00
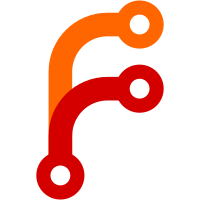
We're not replacing `Path` in exposed definitions in many cases, but just adding alternatives. This will allow us to "top down" change `Path` to `std::fileysystem::path`, and then we can remove the `Path`-using utilities which will become unused. Also add some test files which we forgot to include in the libutil unit tests `meson.build`. Co-Authored-By: siddhantCodes <siddhantk232@gmail.com>
52 lines
1 KiB
C++
52 lines
1 KiB
C++
#pragma once
|
|
///@file
|
|
|
|
#include <optional>
|
|
#include <string>
|
|
#include <string_view>
|
|
|
|
namespace nix {
|
|
|
|
/**
|
|
* Named because it is similar to the Rust type, except it is in the
|
|
* native encoding not WTF-8.
|
|
*
|
|
* Same as `std::filesystem::path::value_type`, but manually defined to
|
|
* avoid including a much more complex header.
|
|
*/
|
|
using OsChar =
|
|
#if defined(_WIN32) && !defined(__CYGWIN__)
|
|
wchar_t
|
|
#else
|
|
char
|
|
#endif
|
|
;
|
|
|
|
/**
|
|
* Named because it is similar to the Rust type, except it is in the
|
|
* native encoding not WTF-8.
|
|
*
|
|
* Same as `std::filesystem::path::string_type`, but manually defined
|
|
* for the same reason as `OsChar`.
|
|
*/
|
|
using OsString = std::basic_string<OsChar>;
|
|
|
|
/**
|
|
* `std::string_view` counterpart for `OsString`.
|
|
*/
|
|
using OsStringView = std::basic_string_view<OsChar>;
|
|
|
|
std::string os_string_to_string(OsStringView path);
|
|
|
|
OsString string_to_os_string(std::string_view s);
|
|
|
|
/**
|
|
* Create string literals with the native character width of paths
|
|
*/
|
|
#ifndef _WIN32
|
|
# define OS_STR(s) s
|
|
#else
|
|
# define OS_STR(s) L##s
|
|
#endif
|
|
|
|
}
|