mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-11-15 18:56:16 +02:00
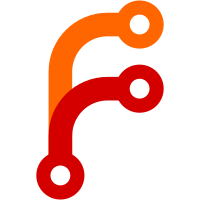
To avoid dealing with an optional `drvPath` (because we might not know it yet) everywhere, make an `CreateDerivationAndRealiseGoal`. This goal just builds/substitutes the derivation file, and then kicks of a build for that obtained derivation; in other words it does the chaining of goals when the drv file is missing (as can already be the case) or computed (new case). This also means the `getDerivation` state can be removed from `DerivationGoal`, which makes the `BasicDerivation` / in memory case and `Derivation` / drv file file case closer together. The map type is factored out for clarity, and because we will soon hvae a second use for it (`Derivation` itself). Co-authored-by: Robert Hensing <roberth@users.noreply.github.com>
125 lines
2.7 KiB
C++
125 lines
2.7 KiB
C++
#pragma once
|
|
///@file
|
|
|
|
#include "lock.hh"
|
|
#include "store-api.hh"
|
|
#include "goal.hh"
|
|
|
|
namespace nix {
|
|
|
|
class Worker;
|
|
|
|
struct PathSubstitutionGoal : public Goal
|
|
{
|
|
/**
|
|
* The store path that should be realised through a substitute.
|
|
*/
|
|
StorePath storePath;
|
|
|
|
/**
|
|
* The path the substituter refers to the path as. This will be
|
|
* different when the stores have different names.
|
|
*/
|
|
std::optional<StorePath> subPath;
|
|
|
|
/**
|
|
* The remaining substituters.
|
|
*/
|
|
std::list<ref<Store>> subs;
|
|
|
|
/**
|
|
* The current substituter.
|
|
*/
|
|
std::shared_ptr<Store> sub;
|
|
|
|
/**
|
|
* Whether a substituter failed.
|
|
*/
|
|
bool substituterFailed = false;
|
|
|
|
/**
|
|
* Path info returned by the substituter's query info operation.
|
|
*/
|
|
std::shared_ptr<const ValidPathInfo> info;
|
|
|
|
/**
|
|
* Pipe for the substituter's standard output.
|
|
*/
|
|
Pipe outPipe;
|
|
|
|
/**
|
|
* The substituter thread.
|
|
*/
|
|
std::thread thr;
|
|
|
|
std::promise<void> promise;
|
|
|
|
/**
|
|
* Whether to try to repair a valid path.
|
|
*/
|
|
RepairFlag repair;
|
|
|
|
/**
|
|
* Location where we're downloading the substitute. Differs from
|
|
* storePath when doing a repair.
|
|
*/
|
|
Path destPath;
|
|
|
|
std::unique_ptr<MaintainCount<uint64_t>> maintainExpectedSubstitutions,
|
|
maintainRunningSubstitutions, maintainExpectedNar, maintainExpectedDownload;
|
|
|
|
typedef void (PathSubstitutionGoal::*GoalState)();
|
|
GoalState state;
|
|
|
|
/**
|
|
* Content address for recomputing store path
|
|
*/
|
|
std::optional<ContentAddress> ca;
|
|
|
|
void done(
|
|
ExitCode result,
|
|
BuildResult::Status status,
|
|
std::optional<std::string> errorMsg = {});
|
|
|
|
public:
|
|
PathSubstitutionGoal(const StorePath & storePath, Worker & worker, RepairFlag repair = NoRepair, std::optional<ContentAddress> ca = std::nullopt);
|
|
~PathSubstitutionGoal();
|
|
|
|
void timedOut(Error && ex) override { abort(); };
|
|
|
|
/**
|
|
* We prepend "a$" to the key name to ensure substitution goals
|
|
* happen before derivation goals.
|
|
*/
|
|
std::string key() override
|
|
{
|
|
return "a$" + std::string(storePath.name()) + "$" + worker.store.printStorePath(storePath);
|
|
}
|
|
|
|
void work() override;
|
|
|
|
/**
|
|
* The states.
|
|
*/
|
|
void init();
|
|
void tryNext();
|
|
void gotInfo();
|
|
void referencesValid();
|
|
void tryToRun();
|
|
void finished();
|
|
|
|
/**
|
|
* Callback used by the worker to write to the log.
|
|
*/
|
|
void handleChildOutput(int fd, std::string_view data) override;
|
|
void handleEOF(int fd) override;
|
|
|
|
/* Called by destructor, can't be overridden */
|
|
void cleanup() override final;
|
|
|
|
JobCategory jobCategory() const override {
|
|
return JobCategory::Substitution;
|
|
};
|
|
};
|
|
|
|
}
|