mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-09-21 08:58:04 +03:00
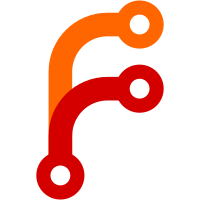
Add a new `file` fetcher type, which will fetch a plain file over http(s), or from the local file. Because plain `http(s)://` or `file://` urls can already correspond to `tarball` inputs (if the path ends-up with a know archive extension), the URL parsing logic is a bit convuluted in that: - {http,https,file}:// urls will be interpreted as either a tarball or a file input, depending on the extensions of the path part (so `https://foo.com/bar` will be a `file` input and `https://foo.com/bar.tar.gz` as a `tarball` input) - `file+{something}://` urls will be interpreted as `file` urls (with the `file+` part removed) - `tarball+{something}://` urls will be interpreted as `tarball` urls (with the `tarball+` part removed) Fix #3785 Co-Authored-By: Tony Olagbaiye <me@fron.io>
46 lines
1.1 KiB
C++
46 lines
1.1 KiB
C++
#pragma once
|
||
|
||
#include "error.hh"
|
||
|
||
namespace nix {
|
||
|
||
struct ParsedURL
|
||
{
|
||
std::string url;
|
||
std::string base; // URL without query/fragment
|
||
std::string scheme;
|
||
std::optional<std::string> authority;
|
||
std::string path;
|
||
std::map<std::string, std::string> query;
|
||
std::string fragment;
|
||
|
||
std::string to_string() const;
|
||
|
||
bool operator ==(const ParsedURL & other) const;
|
||
};
|
||
|
||
MakeError(BadURL, Error);
|
||
|
||
std::string percentDecode(std::string_view in);
|
||
|
||
std::map<std::string, std::string> decodeQuery(const std::string & query);
|
||
|
||
ParsedURL parseURL(const std::string & url);
|
||
|
||
/*
|
||
* Although that’s not really standardized anywhere, an number of tools
|
||
* use a scheme of the form 'x+y' in urls, where y is the “transport layer”
|
||
* scheme, and x is the “application layer” scheme.
|
||
*
|
||
* For example git uses `git+https` to designate remotes using a Git
|
||
* protocol over http.
|
||
*/
|
||
struct ParsedUrlScheme {
|
||
std::optional<std::string_view> application;
|
||
std::string_view transport;
|
||
};
|
||
|
||
ParsedUrlScheme parseUrlScheme(std::string_view scheme);
|
||
|
||
}
|