mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-09-22 01:18:03 +03:00
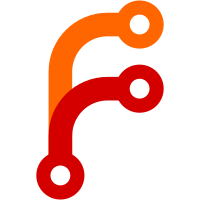
The attributes previously stored in TreeInfo (narHash, revCount, lastModified) are now stored in Input. This makes it less arbitrary what attributes are stored where. As a result, the lock file format has changed. An entry like "info": { "lastModified": 1585405475, "narHash": "sha256-bESW0n4KgPmZ0luxvwJ+UyATrC6iIltVCsGdLiphVeE=" }, "locked": { "owner": "NixOS", "repo": "nixpkgs", "rev": "b88ff468e9850410070d4e0ccd68c7011f15b2be", "type": "github" }, is now stored as "locked": { "owner": "NixOS", "repo": "nixpkgs", "rev": "b88ff468e9850410070d4e0ccd68c7011f15b2be", "type": "github", "lastModified": 1585405475, "narHash": "sha256-bESW0n4KgPmZ0luxvwJ+UyATrC6iIltVCsGdLiphVeE=" }, The 'Input' class is now a dumb set of attributes. All the fetcher implementations subclass InputScheme, not Input. This simplifies the API. Also, fix substitution of flake inputs. This was broken since lazy flake fetching started using fetchTree internally.
111 lines
3.5 KiB
C++
111 lines
3.5 KiB
C++
#include "fetchers.hh"
|
|
#include "store-api.hh"
|
|
|
|
namespace nix::fetchers {
|
|
|
|
struct PathInputScheme : InputScheme
|
|
{
|
|
std::optional<Input> inputFromURL(const ParsedURL & url) override
|
|
{
|
|
if (url.scheme != "path") return {};
|
|
|
|
if (url.authority && *url.authority != "")
|
|
throw Error("path URL '%s' should not have an authority ('%s')", url.url, *url.authority);
|
|
|
|
Input input;
|
|
input.attrs.insert_or_assign("type", "path");
|
|
input.attrs.insert_or_assign("path", url.path);
|
|
|
|
for (auto & [name, value] : url.query)
|
|
if (name == "rev" || name == "narHash")
|
|
input.attrs.insert_or_assign(name, value);
|
|
else if (name == "revCount" || name == "lastModified") {
|
|
uint64_t n;
|
|
if (!string2Int(value, n))
|
|
throw Error("path URL '%s' has invalid parameter '%s'", url.to_string(), name);
|
|
input.attrs.insert_or_assign(name, n);
|
|
}
|
|
else
|
|
throw Error("path URL '%s' has unsupported parameter '%s'", url.to_string(), name);
|
|
|
|
return input;
|
|
}
|
|
|
|
std::optional<Input> inputFromAttrs(const Attrs & attrs) override
|
|
{
|
|
if (maybeGetStrAttr(attrs, "type") != "path") return {};
|
|
|
|
getStrAttr(attrs, "path");
|
|
|
|
for (auto & [name, value] : attrs)
|
|
/* Allow the user to pass in "fake" tree info
|
|
attributes. This is useful for making a pinned tree
|
|
work the same as the repository from which is exported
|
|
(e.g. path:/nix/store/...-source?lastModified=1585388205&rev=b0c285...). */
|
|
if (name == "type" || name == "rev" || name == "revCount" || name == "lastModified" || name == "narHash" || name == "path")
|
|
// checked in Input::fromAttrs
|
|
;
|
|
else
|
|
throw Error("unsupported path input attribute '%s'", name);
|
|
|
|
Input input;
|
|
input.attrs = attrs;
|
|
return input;
|
|
}
|
|
|
|
ParsedURL toURL(const Input & input) override
|
|
{
|
|
auto query = attrsToQuery(input.attrs);
|
|
query.erase("path");
|
|
query.erase("type");
|
|
return ParsedURL {
|
|
.scheme = "path",
|
|
.path = getStrAttr(input.attrs, "path"),
|
|
.query = query,
|
|
};
|
|
}
|
|
|
|
bool hasAllInfo(const Input & input) override
|
|
{
|
|
return true;
|
|
}
|
|
|
|
std::optional<Path> getSourcePath(const Input & input) override
|
|
{
|
|
return getStrAttr(input.attrs, "path");
|
|
}
|
|
|
|
void markChangedFile(const Input & input, std::string_view file, std::optional<std::string> commitMsg) override
|
|
{
|
|
// nothing to do
|
|
}
|
|
|
|
std::pair<Tree, Input> fetch(ref<Store> store, const Input & input) override
|
|
{
|
|
auto path = getStrAttr(input.attrs, "path");
|
|
|
|
// FIXME: check whether access to 'path' is allowed.
|
|
|
|
auto storePath = store->maybeParseStorePath(path);
|
|
|
|
if (storePath)
|
|
store->addTempRoot(*storePath);
|
|
|
|
if (!storePath || storePath->name() != "source" || !store->isValidPath(*storePath))
|
|
// FIXME: try to substitute storePath.
|
|
storePath = store->addToStore("source", path);
|
|
|
|
return {
|
|
Tree {
|
|
.actualPath = store->toRealPath(*storePath),
|
|
.storePath = std::move(*storePath),
|
|
},
|
|
input
|
|
};
|
|
}
|
|
};
|
|
|
|
static auto r1 = OnStartup([] { registerInputScheme(std::make_unique<PathInputScheme>()); });
|
|
|
|
}
|