mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-11-10 16:26:18 +02:00
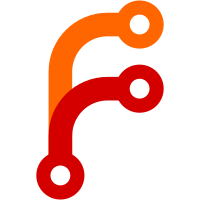
Unlike "nix-store --verify-path", this command verifies signatures in addition to store path contents, is multi-threaded (especially useful when verifying binary caches), and has a progress indicator. Example use: $ nix verify-paths --store https://cache.nixos.org -r $(type -p thunderbird) ... [17/132 checked] checking ‘/nix/store/rawakphadqrqxr6zri2rmnxh03gqkrl3-autogen-5.18.6’
93 lines
2.4 KiB
C++
93 lines
2.4 KiB
C++
#include "command.hh"
|
||
#include "store-api.hh"
|
||
|
||
namespace nix {
|
||
|
||
Commands * RegisterCommand::commands = 0;
|
||
|
||
MultiCommand::MultiCommand(const Commands & _commands)
|
||
: commands(_commands)
|
||
{
|
||
expectedArgs.push_back(ExpectedArg{"command", 1, [=](Strings ss) {
|
||
assert(!command);
|
||
auto i = commands.find(ss.front());
|
||
if (i == commands.end())
|
||
throw UsageError(format("‘%1%’ is not a recognised command") % ss.front());
|
||
command = i->second;
|
||
}});
|
||
}
|
||
|
||
void MultiCommand::printHelp(const string & programName, std::ostream & out)
|
||
{
|
||
if (command) {
|
||
command->printHelp(programName + " " + command->name(), out);
|
||
return;
|
||
}
|
||
|
||
out << "Usage: " << programName << " <COMMAND> <FLAGS>... <ARGS>...\n";
|
||
|
||
out << "\n";
|
||
out << "Common flags:\n";
|
||
printFlags(out);
|
||
|
||
out << "\n";
|
||
out << "Available commands:\n";
|
||
|
||
Table2 table;
|
||
for (auto & command : commands)
|
||
table.push_back(std::make_pair(command.second->name(), command.second->description()));
|
||
printTable(out, table);
|
||
|
||
out << "\n";
|
||
out << "For full documentation, run ‘man " << programName << "’ or ‘man " << programName << "-<COMMAND>’.\n";
|
||
}
|
||
|
||
bool MultiCommand::processFlag(Strings::iterator & pos, Strings::iterator end)
|
||
{
|
||
if (Args::processFlag(pos, end)) return true;
|
||
if (command && command->processFlag(pos, end)) return true;
|
||
return false;
|
||
}
|
||
|
||
bool MultiCommand::processArgs(const Strings & args, bool finish)
|
||
{
|
||
if (command)
|
||
return command->processArgs(args, finish);
|
||
else
|
||
return Args::processArgs(args, finish);
|
||
}
|
||
|
||
StoreCommand::StoreCommand()
|
||
{
|
||
storeUri = getEnv("NIX_REMOTE");
|
||
|
||
mkFlag(0, "store", "store-uri", "URI of the Nix store to use", &storeUri);
|
||
}
|
||
|
||
void StoreCommand::run()
|
||
{
|
||
run(openStoreAt(storeUri));
|
||
}
|
||
|
||
StorePathsCommand::StorePathsCommand()
|
||
{
|
||
expectArgs("paths", &storePaths);
|
||
mkFlag('r', "recursive", "apply operation to closure of the specified paths", &recursive);
|
||
}
|
||
|
||
void StorePathsCommand::run(ref<Store> store)
|
||
{
|
||
for (auto & storePath : storePaths)
|
||
storePath = followLinksToStorePath(storePath);
|
||
|
||
if (recursive) {
|
||
PathSet closure;
|
||
for (auto & storePath : storePaths)
|
||
store->computeFSClosure(storePath, closure, false, false);
|
||
storePaths = store->topoSortPaths(closure);
|
||
}
|
||
|
||
run(store, storePaths);
|
||
}
|
||
|
||
}
|