mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-09-21 17:08:04 +03:00
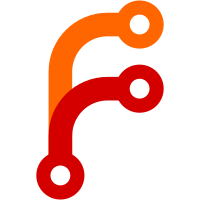
Pos objects are somewhat wasteful as they duplicate the origin file name and input type for each object. on files that produce more than one Pos when parsed this a sizeable waste of memory (one pointer per Pos). the same goes for ptr<Pos> on 64 bit machines: parsing enough source to require 8 bytes to locate a position would need at least 8GB of input and 64GB of expression memory. it's not likely that we'll hit that any time soon, so we can use a uint32_t index to locate positions instead.
47 lines
1.2 KiB
C++
47 lines
1.2 KiB
C++
#pragma once
|
|
|
|
#include "eval.hh"
|
|
|
|
#include <tuple>
|
|
#include <vector>
|
|
|
|
namespace nix {
|
|
|
|
struct RegisterPrimOp
|
|
{
|
|
struct Info
|
|
{
|
|
std::string name;
|
|
std::vector<std::string> args;
|
|
size_t arity = 0;
|
|
const char * doc;
|
|
PrimOpFun fun;
|
|
std::optional<ExperimentalFeature> experimentalFeature;
|
|
};
|
|
|
|
typedef std::vector<Info> PrimOps;
|
|
static PrimOps * primOps;
|
|
|
|
/* You can register a constant by passing an arity of 0. fun
|
|
will get called during EvalState initialization, so there
|
|
may be primops not yet added and builtins is not yet sorted. */
|
|
RegisterPrimOp(
|
|
std::string name,
|
|
size_t arity,
|
|
PrimOpFun fun);
|
|
|
|
RegisterPrimOp(Info && info);
|
|
};
|
|
|
|
/* These primops are disabled without enableNativeCode, but plugins
|
|
may wish to use them in limited contexts without globally enabling
|
|
them. */
|
|
|
|
/* Load a ValueInitializer from a DSO and return whatever it initializes */
|
|
void prim_importNative(EvalState & state, const PosIdx pos, Value * * args, Value & v);
|
|
|
|
/* Execute a program and parse its output */
|
|
void prim_exec(EvalState & state, const PosIdx pos, Value * * args, Value & v);
|
|
|
|
}
|