mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-11-15 18:56:16 +02:00
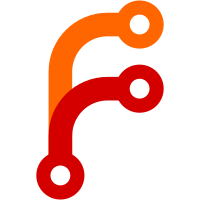
Windows now has some basic Unix Domain Socket support, see https://devblogs.microsoft.com/commandline/af_unix-comes-to-windows/ Building `nix daemon` on Windows I've left for later, because the daemon currently forks per connection but this is not an option on Windows. But we can get the client part working right away.
97 lines
1.9 KiB
C++
97 lines
1.9 KiB
C++
#include "uds-remote-store.hh"
|
|
#include "unix-domain-socket.hh"
|
|
#include "worker-protocol.hh"
|
|
|
|
#include <cstring>
|
|
#include <sys/types.h>
|
|
#include <sys/stat.h>
|
|
#include <errno.h>
|
|
#include <fcntl.h>
|
|
#include <unistd.h>
|
|
|
|
#ifdef _WIN32
|
|
# include <winsock2.h>
|
|
# include <afunix.h>
|
|
#else
|
|
# include <sys/socket.h>
|
|
# include <sys/un.h>
|
|
#endif
|
|
|
|
namespace nix {
|
|
|
|
std::string UDSRemoteStoreConfig::doc()
|
|
{
|
|
return
|
|
#include "uds-remote-store.md"
|
|
;
|
|
}
|
|
|
|
|
|
UDSRemoteStore::UDSRemoteStore(const Params & params)
|
|
: StoreConfig(params)
|
|
, LocalFSStoreConfig(params)
|
|
, RemoteStoreConfig(params)
|
|
, UDSRemoteStoreConfig(params)
|
|
, Store(params)
|
|
, LocalFSStore(params)
|
|
, RemoteStore(params)
|
|
{
|
|
}
|
|
|
|
|
|
UDSRemoteStore::UDSRemoteStore(
|
|
const std::string scheme,
|
|
std::string socket_path,
|
|
const Params & params)
|
|
: UDSRemoteStore(params)
|
|
{
|
|
path.emplace(socket_path);
|
|
}
|
|
|
|
|
|
std::string UDSRemoteStore::getUri()
|
|
{
|
|
if (path) {
|
|
return std::string("unix://") + *path;
|
|
} else {
|
|
return "daemon";
|
|
}
|
|
}
|
|
|
|
|
|
void UDSRemoteStore::Connection::closeWrite()
|
|
{
|
|
shutdown(toSocket(fd.get()), SHUT_WR);
|
|
}
|
|
|
|
|
|
ref<RemoteStore::Connection> UDSRemoteStore::openConnection()
|
|
{
|
|
auto conn = make_ref<Connection>();
|
|
|
|
/* Connect to a daemon that does the privileged work for us. */
|
|
conn->fd = createUnixDomainSocket();
|
|
|
|
nix::connect(toSocket(conn->fd.get()), path ? *path : settings.nixDaemonSocketFile);
|
|
|
|
conn->from.fd = conn->fd.get();
|
|
conn->to.fd = conn->fd.get();
|
|
|
|
conn->startTime = std::chrono::steady_clock::now();
|
|
|
|
return conn;
|
|
}
|
|
|
|
|
|
void UDSRemoteStore::addIndirectRoot(const Path & path)
|
|
{
|
|
auto conn(getConnection());
|
|
conn->to << WorkerProto::Op::AddIndirectRoot << path;
|
|
conn.processStderr();
|
|
readInt(conn->from);
|
|
}
|
|
|
|
|
|
static RegisterStoreImplementation<UDSRemoteStore, UDSRemoteStoreConfig> regUDSRemoteStore;
|
|
|
|
}
|