mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-11-15 18:56:16 +02:00
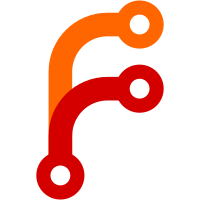
`Store::pathInfoToJSON` was a rather baroque functions, being full of parameters to support both parsed derivations and `nix path-info`. The common core of each, a simple `dValidPathInfo::toJSON` function, is factored out, but the rest of the logic is just duplicated and then specialized to its use-case (at which point it is no longer that duplicated). This keeps the human oriented CLI logic (which is currently unstable) and the core domain logic (export reference graphs with structured attrs, which is stable), separate, which I think is better.
246 lines
6.7 KiB
C++
246 lines
6.7 KiB
C++
#include <nlohmann/json.hpp>
|
|
|
|
#include "path-info.hh"
|
|
#include "store-api.hh"
|
|
#include "json-utils.hh"
|
|
|
|
namespace nix {
|
|
|
|
GENERATE_CMP_EXT(
|
|
,
|
|
UnkeyedValidPathInfo,
|
|
me->deriver,
|
|
me->narHash,
|
|
me->references,
|
|
me->registrationTime,
|
|
me->narSize,
|
|
//me->id,
|
|
me->ultimate,
|
|
me->sigs,
|
|
me->ca);
|
|
|
|
GENERATE_CMP_EXT(
|
|
,
|
|
ValidPathInfo,
|
|
me->path,
|
|
static_cast<const UnkeyedValidPathInfo &>(*me));
|
|
|
|
std::string ValidPathInfo::fingerprint(const Store & store) const
|
|
{
|
|
if (narSize == 0)
|
|
throw Error("cannot calculate fingerprint of path '%s' because its size is not known",
|
|
store.printStorePath(path));
|
|
return
|
|
"1;" + store.printStorePath(path) + ";"
|
|
+ narHash.to_string(HashFormat::Base32, true) + ";"
|
|
+ std::to_string(narSize) + ";"
|
|
+ concatStringsSep(",", store.printStorePathSet(references));
|
|
}
|
|
|
|
|
|
void ValidPathInfo::sign(const Store & store, const SecretKey & secretKey)
|
|
{
|
|
sigs.insert(secretKey.signDetached(fingerprint(store)));
|
|
}
|
|
|
|
std::optional<ContentAddressWithReferences> ValidPathInfo::contentAddressWithReferences() const
|
|
{
|
|
if (! ca)
|
|
return std::nullopt;
|
|
|
|
return std::visit(overloaded {
|
|
[&](const TextIngestionMethod &) -> ContentAddressWithReferences {
|
|
assert(references.count(path) == 0);
|
|
return TextInfo {
|
|
.hash = ca->hash,
|
|
.references = references,
|
|
};
|
|
},
|
|
[&](const FileIngestionMethod & m2) -> ContentAddressWithReferences {
|
|
auto refs = references;
|
|
bool hasSelfReference = false;
|
|
if (refs.count(path)) {
|
|
hasSelfReference = true;
|
|
refs.erase(path);
|
|
}
|
|
return FixedOutputInfo {
|
|
.method = m2,
|
|
.hash = ca->hash,
|
|
.references = {
|
|
.others = std::move(refs),
|
|
.self = hasSelfReference,
|
|
},
|
|
};
|
|
},
|
|
}, ca->method.raw);
|
|
}
|
|
|
|
bool ValidPathInfo::isContentAddressed(const Store & store) const
|
|
{
|
|
auto fullCaOpt = contentAddressWithReferences();
|
|
|
|
if (! fullCaOpt)
|
|
return false;
|
|
|
|
auto caPath = store.makeFixedOutputPathFromCA(path.name(), *fullCaOpt);
|
|
|
|
bool res = caPath == path;
|
|
|
|
if (!res)
|
|
printError("warning: path '%s' claims to be content-addressed but isn't", store.printStorePath(path));
|
|
|
|
return res;
|
|
}
|
|
|
|
|
|
size_t ValidPathInfo::checkSignatures(const Store & store, const PublicKeys & publicKeys) const
|
|
{
|
|
if (isContentAddressed(store)) return maxSigs;
|
|
|
|
size_t good = 0;
|
|
for (auto & sig : sigs)
|
|
if (checkSignature(store, publicKeys, sig))
|
|
good++;
|
|
return good;
|
|
}
|
|
|
|
|
|
bool ValidPathInfo::checkSignature(const Store & store, const PublicKeys & publicKeys, const std::string & sig) const
|
|
{
|
|
return verifyDetached(fingerprint(store), sig, publicKeys);
|
|
}
|
|
|
|
|
|
Strings ValidPathInfo::shortRefs() const
|
|
{
|
|
Strings refs;
|
|
for (auto & r : references)
|
|
refs.push_back(std::string(r.to_string()));
|
|
return refs;
|
|
}
|
|
|
|
ValidPathInfo::ValidPathInfo(
|
|
const Store & store,
|
|
std::string_view name,
|
|
ContentAddressWithReferences && ca,
|
|
Hash narHash)
|
|
: UnkeyedValidPathInfo(narHash)
|
|
, path(store.makeFixedOutputPathFromCA(name, ca))
|
|
{
|
|
std::visit(overloaded {
|
|
[this](TextInfo && ti) {
|
|
this->references = std::move(ti.references);
|
|
this->ca = ContentAddress {
|
|
.method = TextIngestionMethod {},
|
|
.hash = std::move(ti.hash),
|
|
};
|
|
},
|
|
[this](FixedOutputInfo && foi) {
|
|
this->references = std::move(foi.references.others);
|
|
if (foi.references.self)
|
|
this->references.insert(path);
|
|
this->ca = ContentAddress {
|
|
.method = std::move(foi.method),
|
|
.hash = std::move(foi.hash),
|
|
};
|
|
},
|
|
}, std::move(ca).raw);
|
|
}
|
|
|
|
|
|
nlohmann::json ValidPathInfo::toJSON(
|
|
const Store & store,
|
|
bool includeImpureInfo,
|
|
HashFormat hashFormat) const
|
|
{
|
|
using nlohmann::json;
|
|
|
|
auto jsonObject = json::object();
|
|
|
|
jsonObject["path"] = store.printStorePath(path);
|
|
jsonObject["valid"] = true;
|
|
jsonObject["narHash"] = narHash.to_string(hashFormat, true);
|
|
jsonObject["narSize"] = narSize;
|
|
|
|
{
|
|
auto& jsonRefs = (jsonObject["references"] = json::array());
|
|
for (auto & ref : references)
|
|
jsonRefs.emplace_back(store.printStorePath(ref));
|
|
}
|
|
|
|
if (ca)
|
|
jsonObject["ca"] = renderContentAddress(ca);
|
|
|
|
if (includeImpureInfo) {
|
|
if (deriver)
|
|
jsonObject["deriver"] = store.printStorePath(*deriver);
|
|
|
|
if (registrationTime)
|
|
jsonObject["registrationTime"] = registrationTime;
|
|
|
|
if (ultimate)
|
|
jsonObject["ultimate"] = ultimate;
|
|
|
|
if (!sigs.empty()) {
|
|
for (auto & sig : sigs)
|
|
jsonObject["signatures"].push_back(sig);
|
|
}
|
|
}
|
|
|
|
return jsonObject;
|
|
}
|
|
|
|
ValidPathInfo ValidPathInfo::fromJSON(
|
|
const Store & store,
|
|
const nlohmann::json & json)
|
|
{
|
|
using nlohmann::detail::value_t;
|
|
|
|
ValidPathInfo res {
|
|
StorePath(StorePath::dummy),
|
|
Hash(Hash::dummy),
|
|
};
|
|
|
|
ensureType(json, value_t::object);
|
|
res.path = store.parseStorePath(
|
|
static_cast<const std::string &>(
|
|
ensureType(valueAt(json, "path"), value_t::string)));
|
|
res.narHash = Hash::parseAny(
|
|
static_cast<const std::string &>(
|
|
ensureType(valueAt(json, "narHash"), value_t::string)),
|
|
std::nullopt);
|
|
res.narSize = ensureType(valueAt(json, "narSize"), value_t::number_integer);
|
|
|
|
try {
|
|
auto & references = ensureType(valueAt(json, "references"), value_t::array);
|
|
for (auto & input : references)
|
|
res.references.insert(store.parseStorePath(static_cast<const std::string &>
|
|
(input)));
|
|
} catch (Error & e) {
|
|
e.addTrace({}, "while reading key 'references'");
|
|
throw;
|
|
}
|
|
|
|
if (json.contains("ca"))
|
|
res.ca = ContentAddress::parse(
|
|
static_cast<const std::string &>(
|
|
ensureType(valueAt(json, "ca"), value_t::string)));
|
|
|
|
if (json.contains("deriver"))
|
|
res.deriver = store.parseStorePath(
|
|
static_cast<const std::string &>(
|
|
ensureType(valueAt(json, "deriver"), value_t::string)));
|
|
|
|
if (json.contains("registrationTime"))
|
|
res.registrationTime = ensureType(valueAt(json, "registrationTime"), value_t::number_integer);
|
|
|
|
if (json.contains("ultimate"))
|
|
res.ultimate = ensureType(valueAt(json, "ultimate"), value_t::boolean);
|
|
|
|
if (json.contains("signatures"))
|
|
res.sigs = valueAt(json, "signatures");
|
|
|
|
return res;
|
|
}
|
|
|
|
}
|