mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-09-22 17:28:05 +03:00
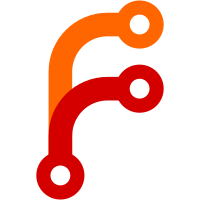
This introduces some shared infrastructure for our notion of protocols. We can then define multiple protocols in terms of that notion. We an also express how particular protocols depend on each other. For example, we can define a common protocol and a worker protocol, where the second depends on the first in terms of the data types it can read and write. The "serve" protocol can just use the common one for now, but will eventually need its own machinary just like the worker protocol for version-aware serialisers
99 lines
2.9 KiB
C++
99 lines
2.9 KiB
C++
#include "serialise.hh"
|
|
#include "util.hh"
|
|
#include "path-with-outputs.hh"
|
|
#include "store-api.hh"
|
|
#include "build-result.hh"
|
|
#include "common-protocol.hh"
|
|
#include "common-protocol-impl.hh"
|
|
#include "archive.hh"
|
|
#include "derivations.hh"
|
|
|
|
#include <nlohmann/json.hpp>
|
|
|
|
namespace nix {
|
|
|
|
/* protocol-agnostic definitions */
|
|
|
|
std::string CommonProto::Serialise<std::string>::read(const Store & store, CommonProto::ReadConn conn)
|
|
{
|
|
return readString(conn.from);
|
|
}
|
|
|
|
void CommonProto::Serialise<std::string>::write(const Store & store, CommonProto::WriteConn conn, const std::string & str)
|
|
{
|
|
conn.to << str;
|
|
}
|
|
|
|
|
|
StorePath CommonProto::Serialise<StorePath>::read(const Store & store, CommonProto::ReadConn conn)
|
|
{
|
|
return store.parseStorePath(readString(conn.from));
|
|
}
|
|
|
|
void CommonProto::Serialise<StorePath>::write(const Store & store, CommonProto::WriteConn conn, const StorePath & storePath)
|
|
{
|
|
conn.to << store.printStorePath(storePath);
|
|
}
|
|
|
|
|
|
ContentAddress CommonProto::Serialise<ContentAddress>::read(const Store & store, CommonProto::ReadConn conn)
|
|
{
|
|
return ContentAddress::parse(readString(conn.from));
|
|
}
|
|
|
|
void CommonProto::Serialise<ContentAddress>::write(const Store & store, CommonProto::WriteConn conn, const ContentAddress & ca)
|
|
{
|
|
conn.to << renderContentAddress(ca);
|
|
}
|
|
|
|
|
|
Realisation CommonProto::Serialise<Realisation>::read(const Store & store, CommonProto::ReadConn conn)
|
|
{
|
|
std::string rawInput = readString(conn.from);
|
|
return Realisation::fromJSON(
|
|
nlohmann::json::parse(rawInput),
|
|
"remote-protocol"
|
|
);
|
|
}
|
|
|
|
void CommonProto::Serialise<Realisation>::write(const Store & store, CommonProto::WriteConn conn, const Realisation & realisation)
|
|
{
|
|
conn.to << realisation.toJSON().dump();
|
|
}
|
|
|
|
|
|
DrvOutput CommonProto::Serialise<DrvOutput>::read(const Store & store, CommonProto::ReadConn conn)
|
|
{
|
|
return DrvOutput::parse(readString(conn.from));
|
|
}
|
|
|
|
void CommonProto::Serialise<DrvOutput>::write(const Store & store, CommonProto::WriteConn conn, const DrvOutput & drvOutput)
|
|
{
|
|
conn.to << drvOutput.to_string();
|
|
}
|
|
|
|
|
|
std::optional<StorePath> CommonProto::Serialise<std::optional<StorePath>>::read(const Store & store, CommonProto::ReadConn conn)
|
|
{
|
|
auto s = readString(conn.from);
|
|
return s == "" ? std::optional<StorePath> {} : store.parseStorePath(s);
|
|
}
|
|
|
|
void CommonProto::Serialise<std::optional<StorePath>>::write(const Store & store, CommonProto::WriteConn conn, const std::optional<StorePath> & storePathOpt)
|
|
{
|
|
conn.to << (storePathOpt ? store.printStorePath(*storePathOpt) : "");
|
|
}
|
|
|
|
|
|
std::optional<ContentAddress> CommonProto::Serialise<std::optional<ContentAddress>>::read(const Store & store, CommonProto::ReadConn conn)
|
|
{
|
|
return ContentAddress::parseOpt(readString(conn.from));
|
|
}
|
|
|
|
void CommonProto::Serialise<std::optional<ContentAddress>>::write(const Store & store, CommonProto::WriteConn conn, const std::optional<ContentAddress> & caOpt)
|
|
{
|
|
conn.to << (caOpt ? renderContentAddress(*caOpt) : "");
|
|
}
|
|
|
|
}
|