mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-11-28 16:46:16 +02:00
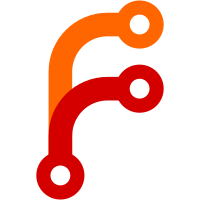
GitArchiveInputScheme now streams tarballs into a Git repository. This deduplicates data a lot, e.g. when you're fetching different revisions of the Nixpkgs repo. It also warns if the tree hash returned by GitHub doesn't match the tree hash of the imported tarball.
44 lines
1.1 KiB
C++
44 lines
1.1 KiB
C++
#pragma once
|
|
///@file
|
|
|
|
#include "types.hh"
|
|
#include "hash.hh"
|
|
|
|
#include <variant>
|
|
|
|
#include <nlohmann/json_fwd.hpp>
|
|
|
|
#include <optional>
|
|
|
|
namespace nix::fetchers {
|
|
|
|
typedef std::variant<std::string, uint64_t, Explicit<bool>> Attr;
|
|
|
|
/**
|
|
* An `Attrs` can be thought of a JSON object restricted or simplified
|
|
* to be "flat", not containing any subcontainers (arrays or objects)
|
|
* and also not containing any `null`s.
|
|
*/
|
|
typedef std::map<std::string, Attr> Attrs;
|
|
|
|
Attrs jsonToAttrs(const nlohmann::json & json);
|
|
|
|
nlohmann::json attrsToJSON(const Attrs & attrs);
|
|
|
|
std::optional<std::string> maybeGetStrAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::string getStrAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::optional<uint64_t> maybeGetIntAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
uint64_t getIntAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::optional<bool> maybeGetBoolAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
bool getBoolAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
std::map<std::string, std::string> attrsToQuery(const Attrs & attrs);
|
|
|
|
Hash getRevAttr(const Attrs & attrs, const std::string & name);
|
|
|
|
}
|