mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-11-10 16:26:18 +02:00
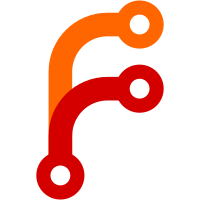
derivation(s) we're interested, e.g., $ nix-instantiate ./all-packages.nix --attr xlibs.libX11 List elements can also be selected: $ nix-instantiate ./build-for-release.nix --attr 0.subversion This allows a non-ambiguous specification of a derivation. Of course, this should also be added to nix-env and nix-build.
65 lines
1.3 KiB
C++
65 lines
1.3 KiB
C++
#ifndef __GET_DRVS_H
|
|
#define __GET_DRVS_H
|
|
|
|
#include <string>
|
|
#include <map>
|
|
|
|
#include "eval.hh"
|
|
|
|
|
|
struct DrvInfo
|
|
{
|
|
private:
|
|
string drvPath;
|
|
string outPath;
|
|
|
|
public:
|
|
string name;
|
|
string system;
|
|
|
|
ATermMap attrs;
|
|
|
|
string queryDrvPath(EvalState & state) const
|
|
{
|
|
if (drvPath == "") {
|
|
Expr a = attrs.get("drvPath");
|
|
(string &) drvPath = a ? evalPath(state, a) : "";
|
|
}
|
|
return drvPath;
|
|
}
|
|
|
|
string queryOutPath(EvalState & state) const
|
|
{
|
|
if (outPath == "") {
|
|
Expr a = attrs.get("outPath");
|
|
if (!a) throw Error("output path missing");
|
|
(string &) outPath = evalPath(state, a);
|
|
}
|
|
return outPath;
|
|
}
|
|
|
|
void setDrvPath(const string & s)
|
|
{
|
|
drvPath = s;
|
|
}
|
|
|
|
void setOutPath(const string & s)
|
|
{
|
|
outPath = s;
|
|
}
|
|
};
|
|
|
|
|
|
typedef list<DrvInfo> DrvInfos;
|
|
|
|
|
|
/* Evaluate expression `e'. If it evaluates to a derivation, store
|
|
information about the derivation in `drv' and return true.
|
|
Otherwise, return false. */
|
|
bool getDerivation(EvalState & state, Expr e, DrvInfo & drv);
|
|
|
|
void getDerivations(EvalState & state, Expr e, DrvInfos & drvs,
|
|
const string & attrPath = "");
|
|
|
|
|
|
#endif /* !__GET_DRVS_H */
|