mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-11-15 18:56:16 +02:00
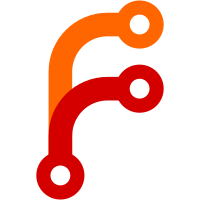
Windows now has some basic Unix Domain Socket support, see https://devblogs.microsoft.com/commandline/af_unix-comes-to-windows/ Building `nix daemon` on Windows I've left for later, because the daemon currently forks per connection but this is not an option on Windows. But we can get the client part working right away.
66 lines
1.7 KiB
C++
66 lines
1.7 KiB
C++
#pragma once
|
|
///@file
|
|
|
|
#include "remote-store.hh"
|
|
#include "remote-store-connection.hh"
|
|
#include "indirect-root-store.hh"
|
|
|
|
namespace nix {
|
|
|
|
struct UDSRemoteStoreConfig : virtual LocalFSStoreConfig, virtual RemoteStoreConfig
|
|
{
|
|
UDSRemoteStoreConfig(const Params & params)
|
|
: StoreConfig(params)
|
|
, LocalFSStoreConfig(params)
|
|
, RemoteStoreConfig(params)
|
|
{
|
|
}
|
|
|
|
const std::string name() override { return "Local Daemon Store"; }
|
|
|
|
std::string doc() override;
|
|
};
|
|
|
|
class UDSRemoteStore : public virtual UDSRemoteStoreConfig
|
|
, public virtual IndirectRootStore
|
|
, public virtual RemoteStore
|
|
{
|
|
public:
|
|
|
|
UDSRemoteStore(const Params & params);
|
|
UDSRemoteStore(const std::string scheme, std::string path, const Params & params);
|
|
|
|
std::string getUri() override;
|
|
|
|
static std::set<std::string> uriSchemes()
|
|
{ return {"unix"}; }
|
|
|
|
ref<SourceAccessor> getFSAccessor(bool requireValidPath = true) override
|
|
{ return LocalFSStore::getFSAccessor(requireValidPath); }
|
|
|
|
void narFromPath(const StorePath & path, Sink & sink) override
|
|
{ LocalFSStore::narFromPath(path, sink); }
|
|
|
|
/**
|
|
* Implementation of `IndirectRootStore::addIndirectRoot()` which
|
|
* delegates to the remote store.
|
|
*
|
|
* The idea is that the client makes the direct symlink, so it is
|
|
* owned managed by the client's user account, and the server makes
|
|
* the indirect symlink.
|
|
*/
|
|
void addIndirectRoot(const Path & path) override;
|
|
|
|
private:
|
|
|
|
struct Connection : RemoteStore::Connection
|
|
{
|
|
AutoCloseFD fd;
|
|
void closeWrite() override;
|
|
};
|
|
|
|
ref<RemoteStore::Connection> openConnection() override;
|
|
std::optional<std::string> path;
|
|
};
|
|
|
|
}
|