mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2025-02-12 13:17:18 +02:00
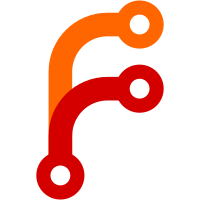
Known behavior changes: - `MemorySourceAccessor`'s comparison operators no longer forget to compare the `SourceAccessor` base class. Progress on #10832 What remains for that issue is hopefully much easier!
66 lines
1.7 KiB
C++
66 lines
1.7 KiB
C++
#include "source-path.hh"
|
|
|
|
namespace nix {
|
|
|
|
std::string_view SourcePath::baseName() const
|
|
{ return path.baseName().value_or("source"); }
|
|
|
|
SourcePath SourcePath::parent() const
|
|
{
|
|
auto p = path.parent();
|
|
assert(p);
|
|
return {accessor, std::move(*p)};
|
|
}
|
|
|
|
std::string SourcePath::readFile() const
|
|
{ return accessor->readFile(path); }
|
|
|
|
bool SourcePath::pathExists() const
|
|
{ return accessor->pathExists(path); }
|
|
|
|
SourceAccessor::Stat SourcePath::lstat() const
|
|
{ return accessor->lstat(path); }
|
|
|
|
std::optional<SourceAccessor::Stat> SourcePath::maybeLstat() const
|
|
{ return accessor->maybeLstat(path); }
|
|
|
|
SourceAccessor::DirEntries SourcePath::readDirectory() const
|
|
{ return accessor->readDirectory(path); }
|
|
|
|
std::string SourcePath::readLink() const
|
|
{ return accessor->readLink(path); }
|
|
|
|
void SourcePath::dumpPath(
|
|
Sink & sink,
|
|
PathFilter & filter) const
|
|
{ return accessor->dumpPath(path, sink, filter); }
|
|
|
|
std::optional<std::filesystem::path> SourcePath::getPhysicalPath() const
|
|
{ return accessor->getPhysicalPath(path); }
|
|
|
|
std::string SourcePath::to_string() const
|
|
{ return accessor->showPath(path); }
|
|
|
|
SourcePath SourcePath::operator / (const CanonPath & x) const
|
|
{ return {accessor, path / x}; }
|
|
|
|
SourcePath SourcePath::operator / (std::string_view c) const
|
|
{ return {accessor, path / c}; }
|
|
|
|
bool SourcePath::operator==(const SourcePath & x) const noexcept
|
|
{
|
|
return std::tie(*accessor, path) == std::tie(*x.accessor, x.path);
|
|
}
|
|
|
|
std::strong_ordering SourcePath::operator<=>(const SourcePath & x) const noexcept
|
|
{
|
|
return std::tie(*accessor, path) <=> std::tie(*x.accessor, x.path);
|
|
}
|
|
|
|
std::ostream & operator<<(std::ostream & str, const SourcePath & path)
|
|
{
|
|
str << path.to_string();
|
|
return str;
|
|
}
|
|
|
|
}
|