mirror of
https://github.com/privatevoid-net/nix-super.git
synced 2024-11-15 18:56:16 +02:00
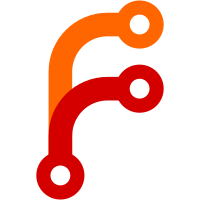
Building derivations is a lot harder, but the downloading goals is portable enough. The "common channel" code is due to Volth. I wonder if there is a way we can factor it out into separate functions / files to avoid some within-function CPP. Co-authored-by: volth <volth@volth.com>
45 lines
781 B
C++
45 lines
781 B
C++
#pragma once
|
|
///@file
|
|
|
|
#include "types.hh"
|
|
|
|
#include <optional>
|
|
|
|
#include <sys/types.h>
|
|
|
|
namespace nix {
|
|
|
|
struct UserLock
|
|
{
|
|
virtual ~UserLock() { }
|
|
|
|
/**
|
|
* Get the first and last UID.
|
|
*/
|
|
std::pair<uid_t, uid_t> getUIDRange()
|
|
{
|
|
auto first = getUID();
|
|
return {first, first + getUIDCount() - 1};
|
|
}
|
|
|
|
/**
|
|
* Get the first UID.
|
|
*/
|
|
virtual uid_t getUID() = 0;
|
|
|
|
virtual uid_t getUIDCount() = 0;
|
|
|
|
virtual gid_t getGID() = 0;
|
|
|
|
virtual std::vector<gid_t> getSupplementaryGIDs() = 0;
|
|
};
|
|
|
|
/**
|
|
* Acquire a user lock for a UID range of size `nrIds`. Note that this
|
|
* may return nullptr if no user is available.
|
|
*/
|
|
std::unique_ptr<UserLock> acquireUserLock(uid_t nrIds, bool useUserNamespace);
|
|
|
|
bool useBuildUsers();
|
|
|
|
}
|